Data structures in Javascript and Python
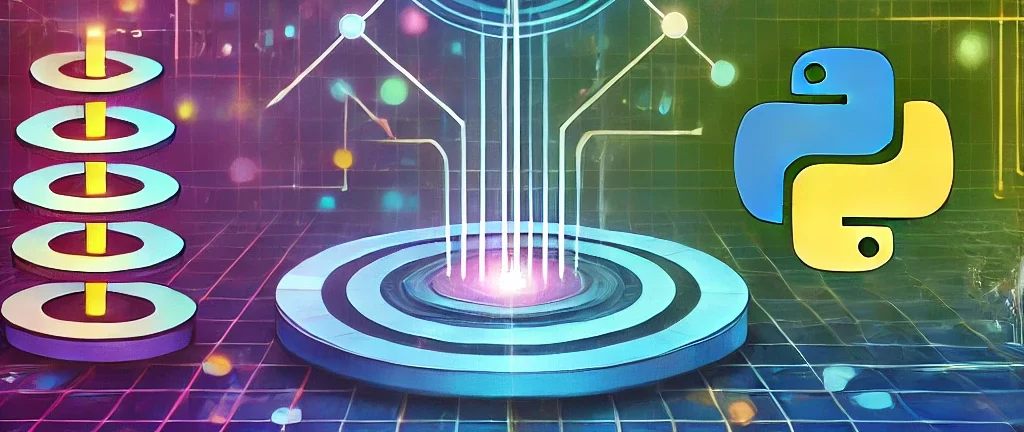
In programming, data structures are essential building blocks for organizing and storing data efficiently. Whether you’re working with a small project or developing large-scale systems, understanding the appropriate data structure to use can significantly improve the performance of your application. In this blog post, we will explore various types of data structures, including arrays, linked lists, stacks, queues, and hash maps, and demonstrate their implementations in both Python and JavaScript. By the end of this post, you’ll have a clear understanding of how to implement these fundamental data structures in two of the most popular programming languages today, empowering you to make informed decisions when choosing the right data structure for your projects.
1. Arrays and Strings
JavaScript
- Array: Implemented using the built-in
Array
object. - String: Strings are immutable sequences of characters.
1 2 3 4 | let arr = [1, 2, 3, 4]; arr.push(5); // Add an element let str = "Hello"; let newStr = str + " World"; // String concatenation |
Python
- List: Equivalent to arrays in JavaScript.
- String: Immutable sequence of characters.
1 2 3 4 | arr = [ 1 , 2 , 3 , 4 ] arr.append( 5 ) # Add an element str = "Hello" new_str = str + " World" # String concatenation |
2. Linked Lists
Javascript
1 2 3 4 5 6 | class ListNode { constructor(val) { this .val = val; this .next = null ; } } |
Python
1 2 3 4 | class ListNode: def __init__( self , val = 0 ): self .val = val self . next = None |
3. Stacks and Queues
JavaScript:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 | class Stack { constructor() { this .items = []; } push(element) { this .items.push(element); } pop() { return this .items.pop(); } } class Queue { constructor() { this .items = []; } enqueue(element) { this .items.push(element); } dequeue() { return this .items.shift(); } } |
Python
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 | class Stack: def __init__( self ): self .items = [] def push( self , item): self .items.append(item) def pop( self ): return self .items.pop() from collections import deque class Queue: def __init__( self ): self .queue = deque() def enqueue( self , item): self .queue.append(item) def dequeue( self ): return self .queue.popleft() |
4. Hash Tables (Hash Maps)
JavaScript
1 2 3 | let hashMap = new Map(); hashMap.set( "key" , "value" ); console.log(hashMap.get( "key" )); |
Python
1 2 3 | hash_map = {} hash_map[ "key" ] = "value" print (hash_map[ "key" ]) |
5. Trees and Graphs
JavaScript
1 2 3 4 5 6 | class TreeNode { constructor(val) { this .val = val; this .left = this .right = null ; } } |
Python
1 2 3 4 | class TreeNode: def __init__( self , val = 0 ): self .val = val self .left = self .right = None |
6. Heaps (Priority Queues)
JavaScript
1 2 3 4 5 6 7 8 9 10 11 12 | class MinHeap { constructor() { this .heap = []; } insert(val) { this .heap.push(val); this .heap.sort((a, b) => a - b); } extractMin() { return this .heap.shift(); } } |
Python
1 2 3 4 5 | import heapq heap = [] heapq.heappush(heap, 5 ) heapq.heappush(heap, 3 ) print (heapq.heappop(heap)) # 3 |
7. Tries (Prefix Trees)
JavaScript
1 2 3 4 5 6 | class TrieNode { constructor() { this .children = {}; this .isEndOfWord = false ; } } |
Python
1 2 3 4 | class TrieNode: def __init__( self ): self .children = {} self .is_end_of_word = False |
In this blog post, we have covered the essential data structures, their theoretical concepts, and practical implementations in both Python and JavaScript. By comparing these implementations, we have gained insights into how different languages offer varying approaches to the same fundamental concepts. Whether you’re optimizing algorithms, improving memory efficiency, or simplifying code, choosing the right data structure is crucial for achieving the best results. We hope this guide serves as a ready to use resource for your future programming endeavors, and encourages you to explore even more advanced data structures and algorithms in your development journey.