Interactive SSH Sessions with Python
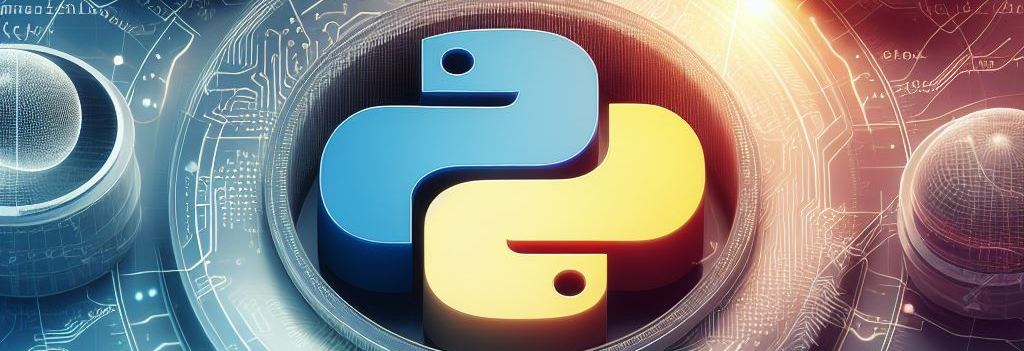
Interactive SSH Sessions with Python: A Guide to Real-Time Command Output
In the world of server management and automation, efficiency is key. Whether you’re a seasoned sysadmin or a budding developer, the ability to execute commands remotely and receive instant feedback can significantly streamline your workflow. This is where Python’s Paramiko library comes into play.
Today, we’re diving into a Python script that not only executes commands via SSH but also streams the output in real-time, giving you a dynamic and responsive shell experience.
Paramiko is a Python module that provides an interface for working with SSH2 protocol. It’s the backbone of many automation scripts that interact with servers and network devices. With Paramiko, you can establish an SSH session, authenticate users, and run commands – all within your Python environment.
The Challenge
A common hurdle when working with remote command execution is the lack of real-time output. Traditional methods often wait for the command to complete before displaying any output, leaving you in the dark about the progress of your command. This can be particularly troublesome when running long or complex scripts.
The solution
Our Python script tackles this challenge head-on and creates interactive SSH Sessions with Python. By leveraging Paramiko’s capabilities, we will craft a solution that stream command output as it happens. This means you’ll see each line of output the moment it’s generated by the server, just as if you were working directly in the terminal.
The script initiates an SSH session using credentials stored in environment variables. It then executes each command and streams the output in real-time.
Interactive SSH Sessions with Python
from paramiko import SSHClient, AutoAddPolicy from os import getenv ssh = SSHClient() ssh.set_missing_host_key_policy(AutoAddPolicy()) # not secure, use with caution in production ssh.connect(hostname=getenv('SSH_HOST'), port=int(getenv('SSH_PORT')), username=getenv('SSH_USER'), password=getenv('SSH_PASSWORD'), look_for_keys=False) # Execute the command _, stdout, stderr = ssh.exec_command(command.format(site)) # Stream stdout while not stdout.channel.exit_status_ready(): # Only print data if there is data to read in the stdout channel if stdout.channel.recv_ready(): rl, _, _ = select.select([stdout.channel], [], [], 0.1) if rl: print(stdout.channel.recv(1024).decode('utf-8'), end='') # Check for any errors error = stderr.read().decode('utf-8') if error: print('STDERR:', error) ssh.close()