Efficiently searching rows by matching key-value pairs in a Jsonb array using sequelize
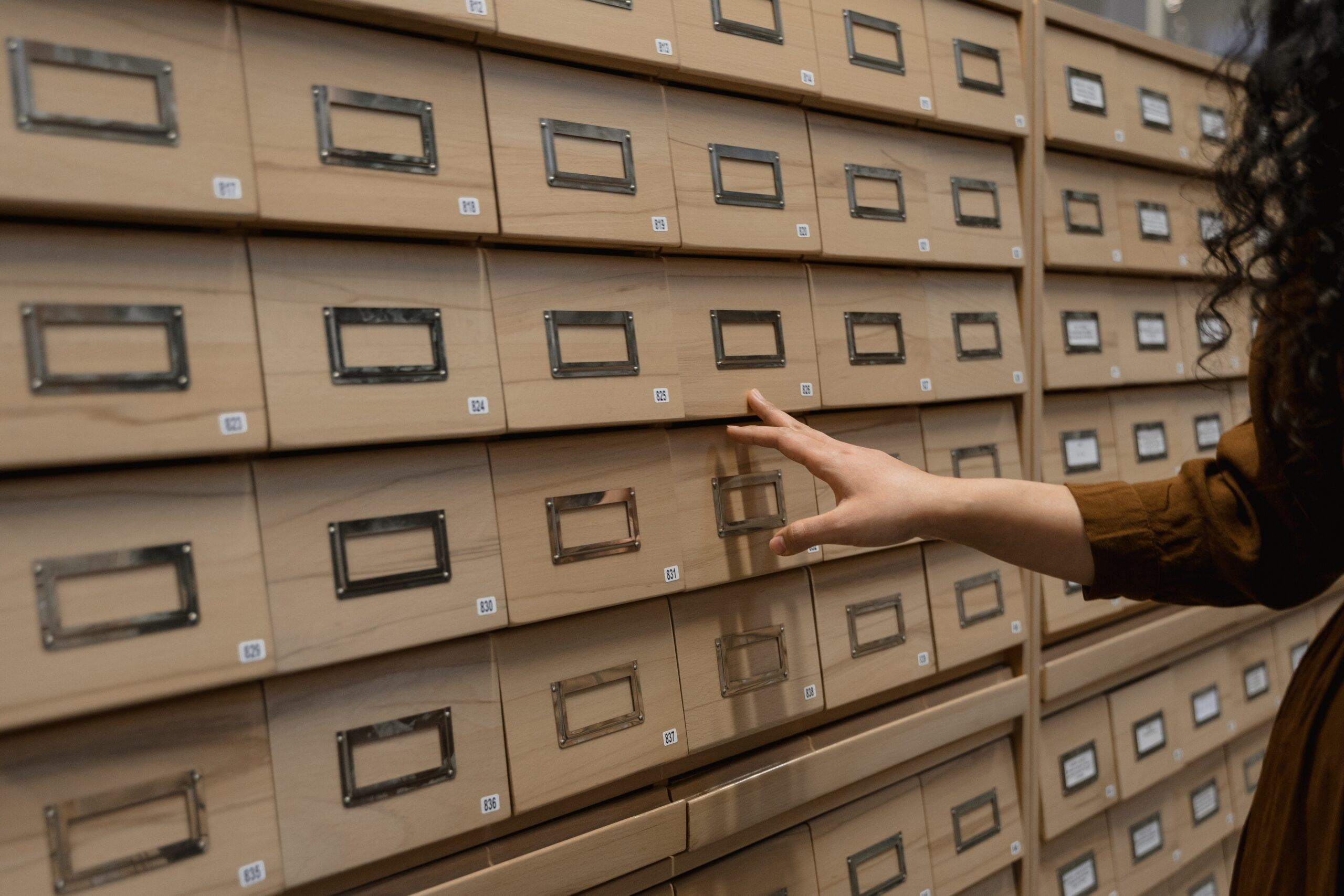
In today’s web development, handling JSON data has become increasingly prevalent, and databases have evolved to accommodate this trend. Sequelize, a widely-used ORM (Object-Relational Mapping) for Node.js, offers robust support for working with JSONB (binary JSON) data types in relational databases. In this blog post, we will explore efficiently searching rows by matching key-value pairs in a Jsonb array using sequelize.
Understanding JSONB in Sequelize:
JSONB is a binary representation of JSON data, enabling efficient storage and querying of JSON-like structures within a relational database. Sequelize empowers developers to seamlessly work with JSONB columns and provides powerful methods for managing JSONB data.
Scenario:
Let’s consider a scenario where we have a table named your_table
with a column called your_column
, which is of type JSONB. Our goal is to retrieve rows from this table based on specific key-value pairs within the your_column
JSONB array.
The SQL Query:
The SQL query we can use in this scenario is
SELECT * FROM your_table WHERE ( your_column @> '[{"key": "value1"}]'::JSONB OR your_column @> '[{"key": "value2"}]'::JSONB );
Step-by-Step Guide to Replicating the Query in Sequelize:
Step 1: Setting Up Sequelize and Your Model:
Ensure that you have Sequelize installed in your Node.js project. Create a Sequelize model that corresponds to the your_table
table in your database, with the your_column
field defined as JSONB.
Step 2: Import Sequelize and Your Model:
Begin by importing Sequelize and your custom-defined model at the beginning of your Node.js file to access Sequelize’s powerful functionalities.
Step 3: Executing the JSONB Query:
Next, we’ll perform the JSONB query using Sequelize. Leveraging the literal()
method, Sequelize allows us to execute raw SQL expressions directly in our queries.
const sequelize = require('sequelize'); const { Op } = sequelize; const findRowsByMatchingJsonbData = async () => { const results = await YourModel.findAll({ where: sequelize.literal(` ( your_column @> '[{"key": "value1"}]'::JSONB OR your_column @> '[{"key": "value2"}]'::JSONB ) `) }); return results; };
In the code above, we utilize sequelize.literal()
to execute the intricate JSONB query.
In this blog post, we delved into efficiently searching for rows in Sequelize by matching key-value pairs within a JSONB array. By gaining a deep understanding of JSONB data types and leveraging Sequelize’s literal()
method, developers can create highly performant applications that harness the full potential of JSON data within relational databases.
Mastering Sequelize’s JSONB query capabilities empowers developers to build dynamic and efficient applications, making it an indispensable tool for handling JSONB data in Node.js projects. As you embrace this powerful feature, you can craft seamless and responsive applications that leverage the full potential of JSON data in a relational database setting.